Continuous servo rotation after few joy pad commands
Forums:
Hy,
I connected an UltraBorg to my ThunderBorg and Pi. At the TB four 12V DC-Motors are attached, at the UB there are 4 MG996R attached. Everything is powered by 6s Lipo and is doing fine.
At my code, when i press a button, the servos are rotating 45 degree forming a circle to spin arround. Another button moves the servos in the initial position to drive straight.
The problem i detected is, after a few commands (pressed more or less fast button 1 and press button 2 to play around and rejioce the working code) to rotate unrotate the servo, one of the servos rotates continuously. It is not every time the same servo to rotate permanent.
I don't know if i reproduce the failure or the failure comes up after a certain number of commands. I can't stop the rotation until i switch off the battery.
Do you have an idea what's wrong with the servo or UB? Otherwise the code runs well.
Thanks in advance for your answer.
- Log in to post comments

piborg
Wed, 05/13/2020 - 21:36
Permalink
Not sure, but might be an out of range problem
It sounds like the servos might be getting a position beyond their movement range occasionally.
If you can attach the code it might be helpful diagnosing the cause.
Canaoz
Thu, 05/14/2020 - 08:44
Permalink
the code
I am not able to copy the code in a good format, can you help me with this too?
Starting with line 187 i set the position with button command defined at line 58 and 59.
The code is the tbJoystick with added ub parts.
I configured the servos with the tuning GUI to set the max and min. To avoid the rotation or reaching max/min position i reduced the set.servopostion(), but this was not helpful.
thank you
piborg
Thu, 05/14/2020 - 11:43
Permalink
Servo behaviour
I cannot see anything wrong with your code, the problem must be elsewhere.
If you open the UltraBorg GUI it should show you where the UltraBorg has set the servo position to:
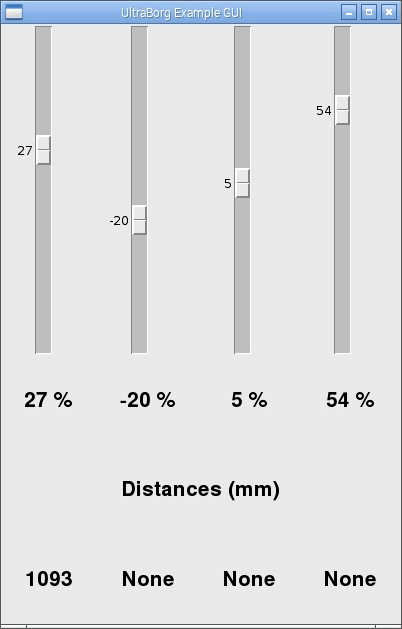
Try moving the sliders up and down to see if you can recreate the problem. If you can then what does the servo position value read for the servo which is constantly rotating?
Canaoz
Fri, 05/15/2020 - 09:00
Permalink
no failure with ubGui
Hy,
i detected no misconduct while playing with the ubGui. I moved the slider up and down or set directly to the min/max position (middle mouse button).
As i played with the joystick, i detected a delay of moving the servos, they move not identical or at the same time. What i think is, there is something wrong with the signals.
The next steps i'll do to detect the cause of the failure are:
- write delay in the code between the sensor moving
- play with 1, 2, 3 numbers of servos to detect at which number of servos the misbehavoiur occurs.
I report the results in the next days.
Nice Weekend to all of you
Canaoz
Mon, 05/18/2020 - 13:37
Permalink
ongoing
Hy,
I made some tests with increasing number of the servos. Up to two servos everything is fine and they move very good. with the third servo the misbehaviours begin. But It is hard to get the failure with 3 servos.
With delay after every servo moving works fine at the first try, but this looks weird and is not really usable. I inserted an single delay after the spin and unspin command. Unfortunately, after several pressed buttons, one of the servos started rotating again directly without counting a delay.
is there another way to configure the speed of the servos as described in the ubSequence.py
Do you have more test in mind i can do?
piborg
Mon, 05/18/2020 - 17:18
Permalink
Servo speed
In the ubSequence.py script we control the speed of the movement by making lots of small moves instead of one big one.
This is fairly easy to implement:
The rate is controlled by both
stepDistance
andstepDelay
. I would recommend reducing the delay to speed things up.The problem is that you will loose any control until the change has finished.
Given the number of servos moving has an effect, this may simply be that the 5V supply for the servos is not able to deliver enough current for all four. What are you using to power the servos?
Canaoz
Wed, 05/20/2020 - 08:36
Permalink
Hy,
Hy,
like in the picture of NASAs clean room, I want to build my own Mars Rover and therefore the front and rear wheels has to move befor changing the direction. Thats why I need the four servo (I know I can spin/steer directly w/o the servo, this was done for introducing myself in the Borgs).
I don't added the speed of the servos like in the ub Sequence, but it will be in the upcoming week.
With a second UB, maybe i can avoid this misbevaiour of the servos too. Can I use one BattBorg for two UB or uses every UB his own BattBorg?
Thank you in advance
Daniel
piborg
Wed, 05/20/2020 - 20:44
Permalink
Fun project
It sounds like a fun project :)
You can power two UltraBorgs from the same BattBorg, they will share the power as needed.
Canaoz
Mon, 05/25/2020 - 08:42
Permalink
fun and challenging
Hy,
yes, the project gives me a lot of fun but also a lot of frustration :D But the finished steps motivates to continue.
I found in the forum (http://forum.piborg.org/node/1812) i can replace the battborg with an UBEC (i have an UBEC-3A-6S and using a 6S-LIPO). Is it this simple to replace the Battborg with the Ubec. The Pi is powered by the connection of the TB. I am using the connection of the picture below.
W.r.t to the connection below. You mentioned i can power two UB with one BattBorg. But for me it is not really clear how to wire. I would just add a connection from V+/ GND of UB1 to V+/ GND to UB2 = parallel connection.
thanks in advance.
piborg
Mon, 05/25/2020 - 11:52
Permalink
Powering servos from a UBEC
Using the UBEC to power the servos is a good idea as it can provide more current for the servos than the BattBorg can.
The first thing to do is ensure both "5V link" jumpers are removed so that the UBEC is not connected to the Pi's 5V line:
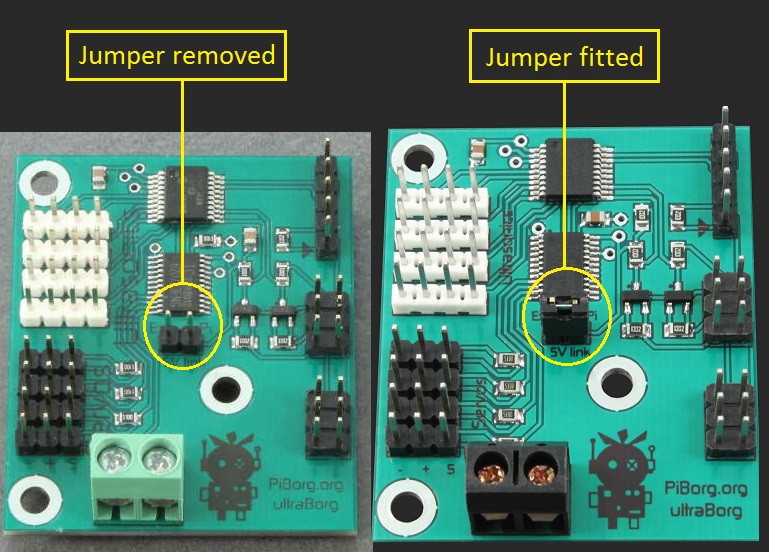
The UBEC then needs to be wired so that the battery + and - are connected to the battery and the output + and - are connected to the V+ and GND screw terminals on both UltraBorgs. These are connected in parallel as you have described (UB1 V+ to UB2 V+, UB1 GND to UB2 GND).
Canaoz
Fri, 05/29/2020 - 15:13
Permalink
UBEC instead of BattBorg
Hy,
I replaced the Battborg with an UBEC to power the UB. I switched many times and very fast my servos with good behaviour, no rotations detected but the yellow flash appeared (with monitor attached to HDMI). I added the 4 motors too and tried again. Still good behaviour and the yellow flash also disappeared.
I am happy and can go one. Add the commands to the webIU and building the chassis.
Thanks for your support.
We read each other :D