LedBorg - An ultra bright RGB LED add on board for your Raspberry Pi
Lesson plan
Installation of the new LedBorg softwareLesson 1. Our first Python example
Lesson 2. Simplifying the code
Lesson 3. Produce a sequence of colours
Lesson 4. Taking user input
Lesson 5. Brightness levels (PWM)
Lesson 6. A simple dialog (GUI)
Lesson 7. Using a pre-made dialog
Produce a sequence of colours
In the previous lesson we saw how we can program the LedBorg to change colours at an interval, but if we wanted to run longer sequences there would still be a lot of typing involved.Surely there is a better way...
It is time to use a list.
Lists in Python
Lists are a way of giving Python a list of values which it can use in a similar way to the variables in the previous example.The biggest difference is that the list can be indexed, this means you can grab a single value from the list using a position number.
This is easier to visualise if we use an example:
data = [1, 2, 3, 5, 8, 13]
In this case data is a list of six values: 1, 2, 3, 5, 8, and 13.
Python indexes starting from zero, this means that
data[0]
is the value 1 and data[3]
is the value 5.We can also put lists into lists:
data = [[1, 2], [2, 4], [3, 6], [5, 10], [8, 16], [13, 26]]
now
data[0]
is the list [1, 2] and data[3]
is the list [5, 10].In Python we can get each value from a list using a for loop.
A for loop is a set of lines which may be used more than once.
In the case of Python the lines inside the for loop are run once for each value in a list.
If we consider the following code:
data = [1, 2, 3, 4] for value in data: print valuethe result we would get printed to the terminal is:
1 2 3 4Each entry from the data list gets given to the value variable in the same order as the list.
Note that the loop has an indented line like the functions from lesson 2, this is because the loop has lines which belong to it and only those lines get run multiple times.
The revised version of the code
We can modify the lesson2.py script to use this new method, start by copying the old script:cp lesson2.py lesson3.py
Then open up the new script in your editor and change lines 26 onwards to match the new version:
#!/usr/bin/env python # Import the library functions we need import time import wiringpi2 as wiringpi wiringpi.wiringPiSetup() # Setup the LedBorg GPIO pins PIN_RED = 0 PIN_GREEN = 2 PIN_BLUE = 3 wiringpi.pinMode(PIN_RED, wiringpi.GPIO.OUTPUT) wiringpi.pinMode(PIN_GREEN, wiringpi.GPIO.OUTPUT) wiringpi.pinMode(PIN_BLUE, wiringpi.GPIO.OUTPUT) # A function to set the LedBorg colours def SetLedBorg(red, green, blue): wiringpi.digitalWrite(PIN_RED, red) wiringpi.digitalWrite(PIN_GREEN, green) wiringpi.digitalWrite(PIN_BLUE, blue) # A function to turn the LedBorg off def LedBorgOff(): SetLedBorg(0, 0, 0) # Settings for the sequence colourSequence = [[1, 0, 0], # Red [0, 1, 0], # Green [0, 0, 1], # Blue [1, 1, 0], # Yellow [0, 1, 1], # Cyan [1, 0, 1], # Magenta [1, 1, 1]] # White delay = 2 # Set the LedBorg to each colour in turn for colour in colourSequence: SetLedBorg(colour[0], colour[1], colour[2]) print 'LedBorg colour:', colour time.sleep(delay) # Turn the LedBorg off LedBorgOff() print 'LedBorg off'The completed script can be downloaded directly to the Raspberry Pi using:
wget -O lesson3.py http://www.piborg.org/downloads/ledborg-new/lesson3.txt
Remember we make the script executable with:
chmod +x lesson3.py
and run it with:
sudo ./lesson3.py
If everything is working you should see the sequence below:
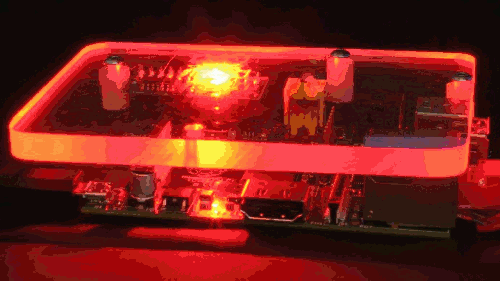
Note a few interesting points here:
- The list of lists is spread across many lines (27-33), this is fine in Python (the indent does not matter in this case)
- There are comments (starting with the
#
symbol) which only use part of the line - The print output is slightly different, it is printing the colour variable instead of a colour name
- The new code is significantly shorter and hopefully easier to understand
Continue to lesson 4. Taking user input
