Replay - Record your LedBorg, then play it back later
We recommend using the new driver free based scripts for LedBorg.
The new driver free examples can be found here, the installation can be found here.
We love to record things, music videos, and now LedBorgs!
It seemed a great idea to be able to record a sequence from one script and play it back by another.
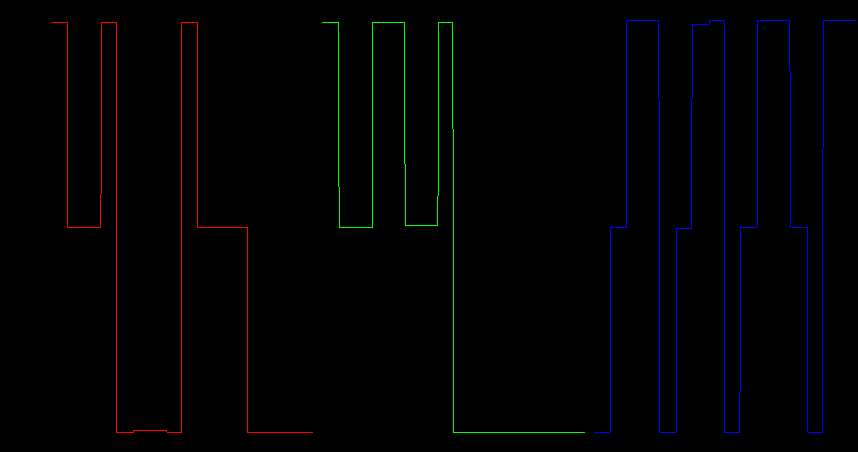
So here we present Replay, our script for recording your LedBorg, and another to play it back later.
Replay comes in two parts:
- ReplayRecord.py
This script records the actions of an attached LedBorg to a named file whilst running - ReplayPlay.py
This script plays back a named recording file at the rate it was recorded
interval
on line 9 of ReplayRecord.py defines the granularity of recording, smaller means a more accurate recording but a larger file is generated.Here's the code, you can download the ReplayRecord script file as text here,
and you can download the ReplayPlay script file as text here.
Save the text files on your Raspberry Pi as ReplayRecord.py and ReplayPlay.py respectively.
Make the scripts executable using
chmod +x Replay*.py
record using
./ReplayRecord.py myvidname
and play using
./ReplayPlay.py myvidname
ReplayRecord
#!/usr/bin/env python # coding: Latin-1 # Load library functions we want import sys import time # Settings for the Replay recorder interval = 0.01 # Number of seconds between updates, smaller makes a more accurate recording but recordings are larger # Get user input if len(sys.argv) > 1: recordPath = sys.argv[1] else: # No file specified, print usage details and exit print 'No file specified for recording, usage:' print '%s fileToRecord' % (sys.argv[0]) sys.exit() # Open the file for recording recordFile = open(recordPath, 'w') print 'Recording to "' + recordPath + '"' print 'Press CTRL+C to end recording' # Function to read the LedBorg colour def GetLedColour(): LedBorg = open('/dev/ledborg', 'r') # Open the LedBorg device for reading from colour = LedBorg.read() # Read the colour string from the LedBorg device LedBorg.close() # Close the LedBorg device return colour # Return the read colour try: # Write the recorded speed at the start of the recording recordFile.write('%f\n' % (interval)) # Loop indefinitely while True: # Get the colour of the LedBorg colour = GetLedColour() # Write the colour to file recordFile.write(colour + '\n') # '\n' is a new line, without this all entries would use the same line # Wait for interval time.sleep(interval) except KeyboardInterrupt: # CTRL+C exit, stop recording and close the file recordFile.close() print 'Recording stopped'
ReplayPlay
#!/usr/bin/env python # coding: Latin-1 # Load library functions we want import sys import time # Get user input if len(sys.argv) > 1: playPath = sys.argv[1] else: # No file specified, print usage details and exit print 'No file specified for playing, usage:' print '%s fileToPlay' % (sys.argv[0]) sys.exit() # Open the file for playing playFile = open(playPath, 'r') print 'Playing from "' + playPath + '"' print 'Press CTRL+C to end playing' # Function to set the LedBorg colour def SetLedColour(colour): LedBorg = open('/dev/ledborg', 'w') # Open the LedBorg device for writing to LedBorg.write(colour) # Write the colour string to the LedBorg device LedBorg.close() # Close the LedBorg device try: # Read the recorded speed at the start of the recording playLine = playFile.readline() interval = float(playLine) # Loop until the file is empty while len(playLine) > 0: # Get the next colour of the LedBorg playLine = playFile.readline() # Set the LedBorg colour SetLedColour(playLine) # Wait for interval time.sleep(interval) # Finished, close the file and switch off the LedBorg playFile.close() SetLedColour('000') print 'Finished' except KeyboardInterrupt: # CTRL+C exit, stop playing and close the file playFile.close() print 'Playing stopped'
