WhatIsYourColour - Show the current LedBorg colour as text
We recommend using the new driver free based scripts for LedBorg.
The new driver free examples can be found here, the installation can be found here.
So you have been playing with some code, and your LedBorg is showing a colour that you like, how would you find out what the colour is called, an HTML colour code, or even just the LedBorg colour code number?
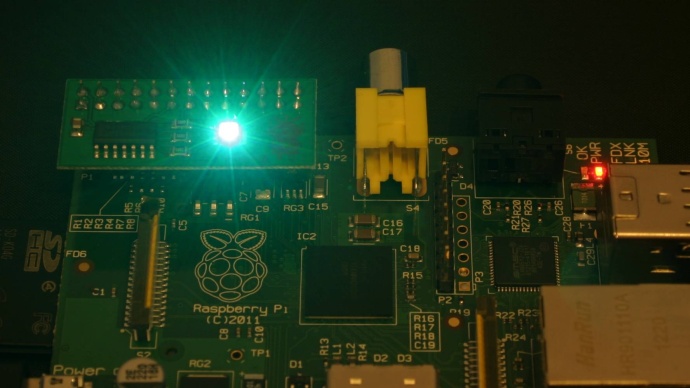
What you need is the inverse of our Swiss Army Led script, a script which reads the LedBorg's current colour and tells you what it is in many different ways:
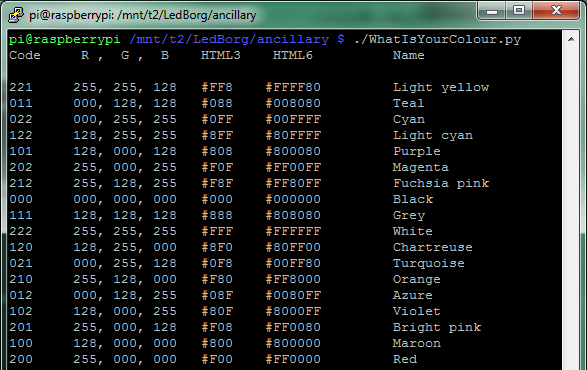
Our answer is to ask the LedBorg "What is your colour?" with a new script called WhatIsYourColour.py
Here's the code, you can download the WhatIsYourColour script file as text here
Save the text file on your pi as WhatIsYourColour.py
Make the script executable using
chmod +x WhatIsYourColour.py
and run using
./WhatIsYourColour.py
#!/usr/bin/env python # coding: Latin-1 # Load library functions we want import time # User settings interval = 1.0 # Number of seconds between readings # Colour codes to names mapping dColourMap = { '100':'Maroon', '200':'Red', '211':'Pink', '110':'Olive', '220':'Yellow', '221':'Light yellow', '010':'Green', '020':'Lime green', '121':'Light green', '011':'Teal', '022':'Cyan', '122':'Light cyan', '001':'Navy', '002':'Blue', '112':'Light blue', '101':'Purple', '202':'Magenta', '212':'Fuchsia pink', '000':'Black', '111':'Grey', '222':'White', '012':'Azure', '102':'Violet', '201':'Bright pink', '120':'Chartreuse', '021':'Turquoise', '210':'Orange' } # Function to work out colour values in 8 bit (0-255) and 4 bit (0-15) def ChannelAs8and4(code): if code == 2: # 2 is fully on, represent as 100% value8 = 255 value4 = 15 elif code == 1: # 1 is half on, represent as ~50% (we will round upwards) value8 = 128 value4 = 8 else: # 0 is off, represent as 0% value8 = 0 value4 = 0 # Return answer as a pair of values return value8, value4 # Display title line print 'Code\t R , G , B \tHTML3\t HTML6\t\tName' print '' # Loop indefinitely while True: # Read the current colour from the LedBorg device LedBorg = open('/dev/ledborg', 'r') # Open the LedBorg device for reading from colourCode = LedBorg.read() # Read the entire contents of the LedBorg file (should only be 3 characters long) LedBorg.close() # Close the LedBorg device red = int(colourCode[0]) # Get the red setting from the colour string green = int(colourCode[1]) # Get the green setting from the colour string blue = int(colourCode[2]) # Get the blue setting from the colour string # Work out the colour name if dColourMap.has_key(colourCode): colourName = dColourMap[colourCode] # Look up the corresponding colour name else: colourName = '???' # Colour code not found # Work out colour values in 8 bit (0-255) and 4 bit (0-15) red8, red4 = ChannelAs8and4(red) green8, green4 = ChannelAs8and4(green) blue8, blue4 = ChannelAs8and4(blue) # Generate 3 and 6 character HTML codes colourHtml3 = '#%01X%01X%01X' % (red4, green4, blue4) colourHtml6 = '#%02X%02X%02X' % (red8, green8, blue8) # Display the full description print '%s\t%03d, %03d, %03d\t%s\t%s\t\t%s' % ( colourCode, red8, green8, blue8, colourHtml3, colourHtml6, colourName) # Wait for the next interval time.sleep(interval)
